Tyreant
tyreant = tyranid + react + ant
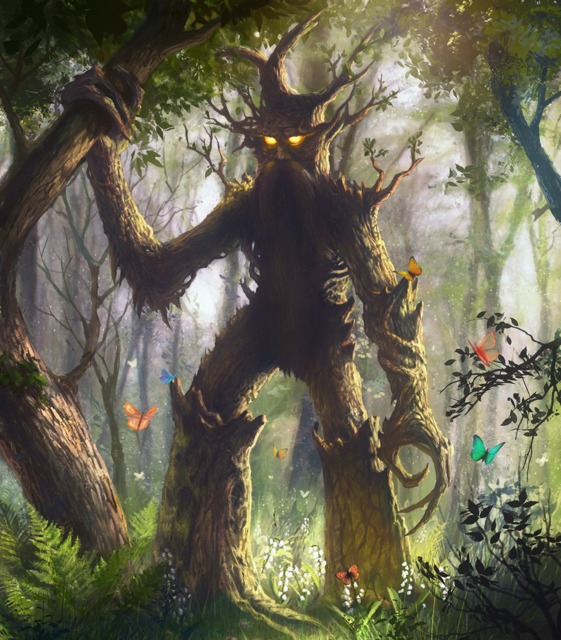
Tyreant is a UI Toolkit library built on top of Tyranid, React, and Ant.
The primary goal behind Tyreant is to create metadata-enabled data-driven UI components.
For example, the following creates a data-driven table that can do its own paging, searching, and so on using the Tyranid metadata and client-side API, along with an edit form:
export const UserTable = () => (
<TyrTable
collection={User},
query={{
status: { $ne: UserStatus.DELETED._id }
}},
columns={[
{ field: 'fullName', defaultSort: 'ascend' },
{ field: 'organizationId' },
{ field: 'email', width: '270px' },
{ field: 'updatedAt', width: '200px' },
{ field: 'status', width: '200px' },
]},
route={'/users'},
actions={[
<TyrFormModal
fields={[
{ field: 'firstName' },
{ field: 'lastName' },
{ field: 'email' },
{ field: 'dateOfBirth' },
{ field: 'status' },
]},
/>,
]}
/>
);
Guiding Principles
- Composable - Multiple components should be able to infer context from other components. For example, a form control nested inside a table control should infer the collection and/or fields from the table and allow the form to be used as an edit form for the table. As another example, Tyreant date fields nested inside a Tyreant form should be able to infer the document they are editing from the form.
- Extensible - Users should be able to write their own Tyreant-compatible, metadata-driven components to extend the component library.
- Intelligent - Components should leverage metadata as much as possible to increase DRYness and provide richer controls.
- Interoperable - Components should be usable on their own alongside non-Tyreant components.
- Offline - Components should operate offline using Tyranid's client-side APIs.
- Performant - Components should be as lightweight as possible and render efficiently.
- Responsive - Components should responsively scale from mobile to desktop.
- Scaleable - Components should be able to have automatic behavior but fallback to more manual react code so that there are no limits to custom behavior. For example, you should be able to specify a form using just a collection and field names or by specifically detailing the field-level components yourself.
- Skinnable - Components should be fully skinnable and styleable and should not lock clients into a particular appearance or style of application.
Roadmap
Completed Roadmap
- TyrLink enhancements
- array of links
- tags
- allow to be nested inside a TyrForm
Immediate Roadmap
- Router plug-in API
- NPM
- Update to latest Ant
- TyrBoolean
- render as two option radio box
- render as checkbox
- TyrInteger / TyrDouble
- support validations
- TyrString
- support regex and length validations
- TyrLink enhancements
- add "details functionality"
- TyrField control enhancements
- placeholder support
- infer document and form from parent TyrForm
- render computed fields dynamically in forms as read-only components
- TyrForm enhancements
- children support
- TyrFormDrawer
- TyrArray control
- TyrTable enhancements
- lift route requirement
- pin columns on the left/right
- show/hide columns
- rearrange columns order
- save and load table configurations/layouts
- multiple selection
- documentation
- layout
- grid layout / side-by-side controls
- autolayout algorithms
- provide ability to estimate "how large" a control will be
- add TyrImage component
- testing framework utilizing puppeteer
Future Roadmap
- TyrPassword
- TyrTable
- column groups
- responsive support
- download
- CSV
- Excel
- pivot table
- mass edit in-place
- TyrAddress/Location
- TyrUnit
- TyrTree
- TyrForm
- multi-step / page forms
- responsive support